Extended explanations
ByVal ByRef, and variables
Part 2 less Simple variable String type
Characters are more complicated for a computer to store, ( https://www.excelfox.com/forum/showt...ll=1#post17877 ) and a lot of text can be extremely long, so desirably we would like this large amount to be available. Currently I think we are talking of sizes around a Giga Bytes or two. But we may not always need this. Unlike in the case of a Long variable type, it would be impractical to routinely set aside a very large amount of memory after/ with a Declaration. More commonly, the address gets assigned once a value is given, ( when the variable gets "filled" ). It is slightly more complicated in the case of VBA as the VBA variable will then contain the address of another pointer which is a specific pointer type, one originating from Visual Basic, sometime referred to as BSTR, (Binary String or Basic String, or a pointer of some sort . Immediately you have opened a can of worms as there is not generical agreement on what a BSTR is). This BSTR (pointer) "goes to" (has the address ("value in it") of), not the start of the memory location as was the case with the Long, but rather to the start of the section containing the representatio0n of the characters of the string. Unlike in the case of the Long, things other than the final value we are interested in ,
_ the a length of the string, at the "left" as it were
and
_ a zero is added at the end
The test coding below used in my explanations is very similar and gives similar results to the previous for the Long case, but there are some subtle things going on that are different: It is very wise therefore to try to understand the Long case from the last post before moving on to here
StrPtr
This is as mysterious as the VarPtr, and was introduced around d the VB4 - VB5 time apparently wired a bit differently to the VarPtr(ByVal ) so as to make sure it went to the correct place, which may somehow help make UNICODE API calls more efficient, since in that use there may be more difficulty in getting the VarPtr(ByVal ) to work as we wish. We expect it to work as the VarPtr(ByVal ) in our experiments
Code:
Sub ByValByRefWithPointersString() ' https://www.excelfox.com/forum/showthread.php/2404-Notes-tests-ByVal-ByRef-Application-Run-OnTime-Multiple-Variable-Arguments-ByRef-ByVal?p=11889&viewfull=1#post11889
1 Dim strOb As String
2 Debug.Print VarPtr(strOb), VarPtr(ByVal strOb), StrPtr(strOb), StrPtr(ByVal strOb) ' 1831204 0 0 0
3 Call ByRefString(strOb) ' Do a ByRef Call --#
7 Debug.Print VarPtr(strOb), VarPtr(ByVal strOb), StrPtr(strOb), StrPtr(ByVal strOb) ' 1831204 86412692 86412692 86412692
8 Let strOb = vbNullString
9 Call ByValString(strOb) ' Do a ByVal Call --**
13 Debug.Print VarPtr(strOb), VarPtr(ByVal strOb), StrPtr(strOb), StrPtr(ByVal strOb) ' 1831204 0 0 0
End Sub
Sub ByRefString(ByRef strObs As String) ' #-- Do a ByRef Call
4 Debug.Print VarPtr(strObs), VarPtr(ByVal strObs), StrPtr(strObs), StrPtr(ByVal strObs) ' 1831204 0 0 0 0 0
5 Let strObs = ""
6 Debug.Print VarPtr(strObs), VarPtr(ByVal strObs), StrPtr(strObs), StrPtr(ByVal strObs) ' 1831204 86412692 86412692 86412692
End Sub
Sub ByValString(ByVal strObs As String) ' **-- Do a ByVal Call
10 Debug.Print VarPtr(strObs), VarPtr(ByVal strObs), StrPtr(strObs), StrPtr(ByVal strObs) ' 1831036 0 0 0
11 Let strObs = ""
12 Debug.Print VarPtr(strObs), VarPtr(ByVal strObs), StrPtr(strObs), StrPtr(ByVal strObs) ' 1831036 86412092 86412092 86412092
End Sub
The first output lines of 2 and 4 give us very similar results to the Long case. The fact that the returned value in both lines are the same demonstrate again that both in the main routine and the Called we are referring to the same variable, which is what the ByRef in the Called Sub ByRefString(ByRef strObs As String) has arranged.
Furthermore, if you run the main coding Sub ByValByRefWithPointersString() on the same computer after running the previous Long case coding from the last post, then , as I did you may get exactly the same results. This is perhaps not too surprising. At this stage we are doing exactly as in the previous coding for the corresponding code lines: At some location not easily known to us, a 32 bit, 4Byte is created holding a number, that number being sometimes regarded as the pointer: it tells us the first "left" of another 4 Bytes of memory location, set aside for us, and they will be initialised to 0 initially, just as previously, as the lines 2 and 4 suggest. (But we are not finished with memory allocation here, as we were in the Ling case!!)
https://i.postimg.cc/2y7gccT9/vb-Null-String-state.jpg
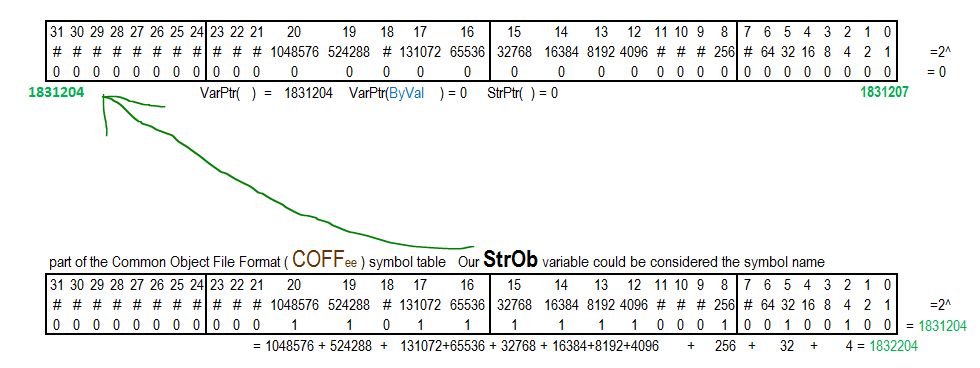
(In passing, note, that this might be considered a "vbNullString situation" , but this is not directly because of the value 0 being held in the above chunk of memory. This 0 value is a consequence of, and an indication of, a vbNullString, but it is not directly depicting in any way a "nothing string" or "not string" or "null string". The specific direct representation is given by the fact that we see nothing else substantial in the picture yet, as we soon will)
Zero length string
In line 5 I deliberately chose the string "" , rather than something like "Alan" , as initially might reasonably have been expected. This is to emphasise that this “„ is not such a direct equivalent to the long of 0. If anything it comes closer to assigning a long value of a moderately large number, similar to the "pointer" values we have discussed in the COFF. In my example I might say that it does something like what Let Lng = 86412692 might have done in the previous coding. But now something else also happens: We get something new, extra, even in the very smallest simplest case of this string "" , which can be considered a string of zero length. A laymen instinct might be to consider 0 and "" as the equivalent things for the appropriate type. But that is more false than true, even if it is not simple black and white.
The following attempts to show this situation, when we "have" , "" , and even in this very smallest simplest case of this string, "" , it is difficult to accurately show the situation in a small simple diagram, because another chunk of memory place is made available, in more typical cases this is much larger than those considered so far. Even for "" , it is larger than those considered so far: For this simplest case it is 6 Bytes, (48 Bits)
Unlike in the case of the Long, the value, in my example here , it is 86412692, (and was 2 for the Long case), is not the final value of interest to me, which in this example is "" , but it is doing a very similar thing to the value of 1831204 in the COFF: It is pointing to this new extra chunk of memory. This "" situation is more commonly called the zero Length string situations
https://i.postimg.cc/8CSsbvrK/Zero-Length-Situation.jpg
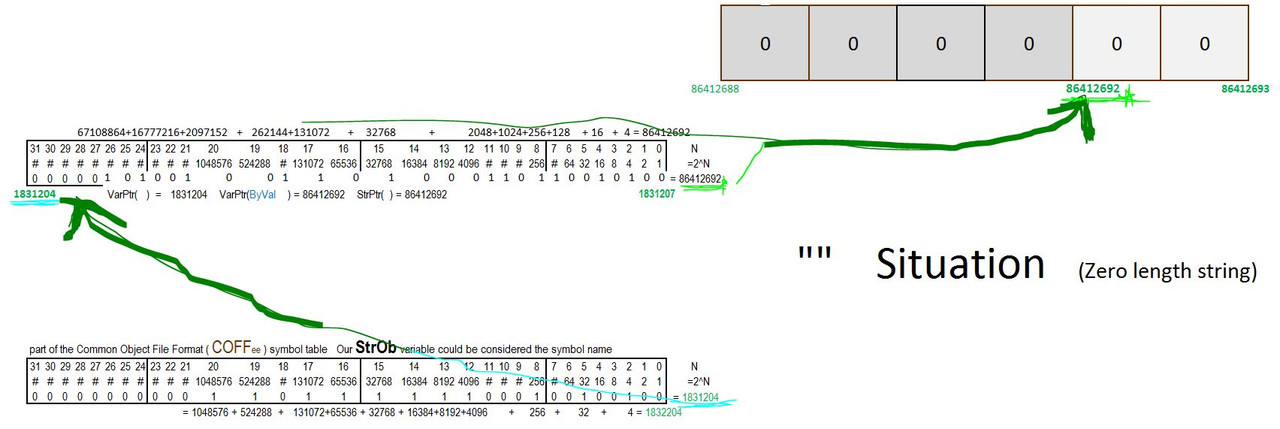
An important observation / concussion here is that the use of = "" does not return us to the state after the Dim, in the same or similar way that the = 0 did/ does in the long case
Lines 6 and 7 are giving the same results, indicating again that we are looking at the same variable in the main routine or the first Called routine, Sub ByRefString(ByRef strObs As String)
_.___________________
Before we take a break, consider what would happen if we simply replaced Let strOb = "" with Let strOb = "A"
Lets zoom in on what the area which would most likely be the only** area changed:
https://i.postimg.cc/BnDm793c/Area-l...assignment.jpg
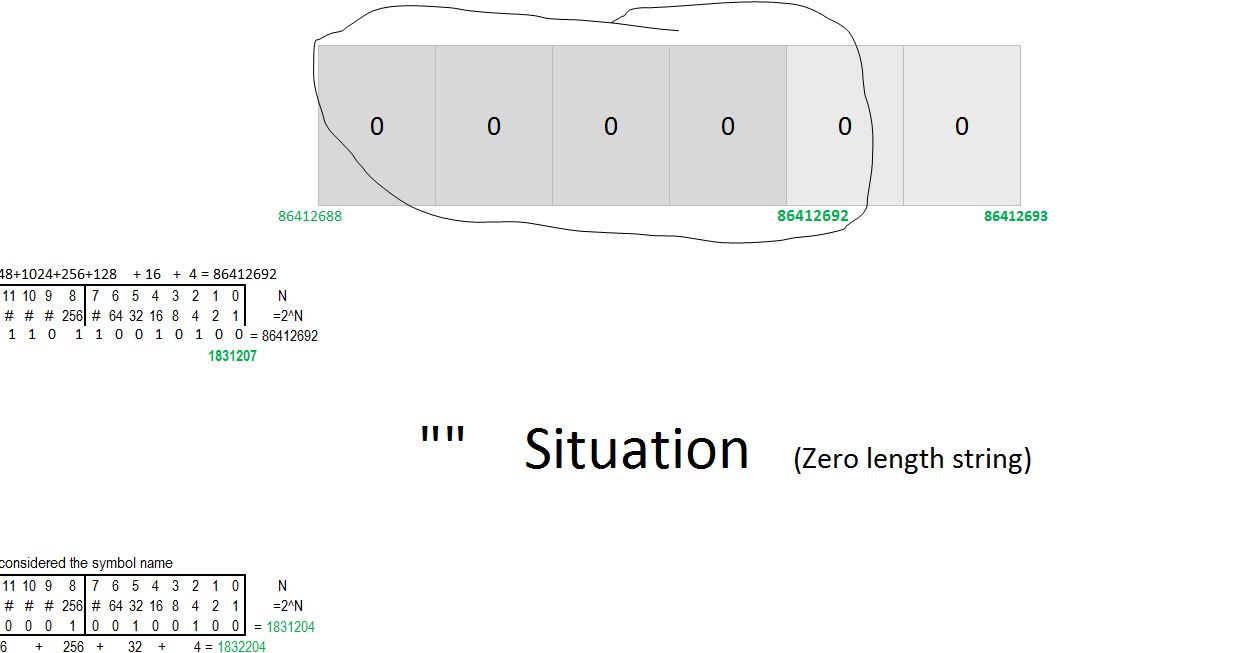
This would most likely be the (only** ) change after replaced Let strOb = "" with Let strOb = "A"
https://i.postimg.cc/8Pxybk2z/Let-str-Ob-A.jpg
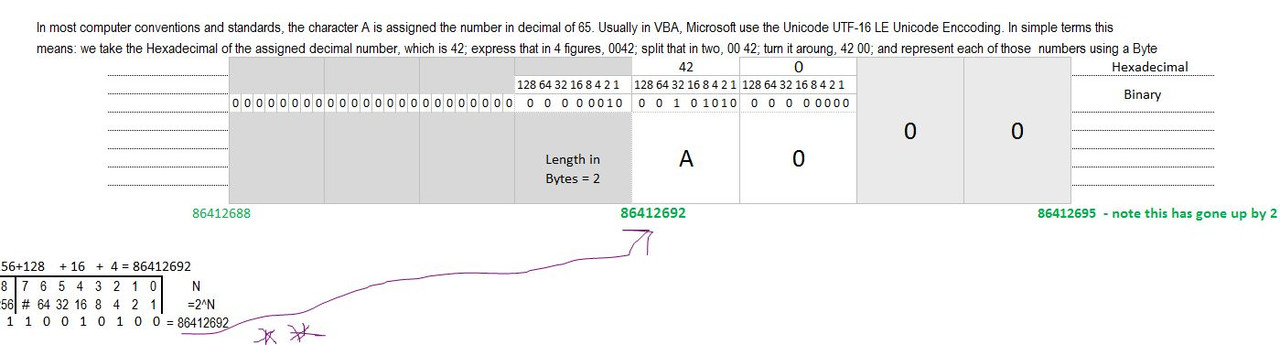
So two extra Bytes are added to represent the character A
_.__
** If you make a substantial change to the length of your character string you may see another change. Consider the following short coding.
Code:
Sub BigStringLength()
Dim strOb As String
Let strOb = ""
Debug.Print VarPtr(strOb), VarPtr(ByVal strOb)
Let strOb = "A"
Debug.Print VarPtr(strOb), VarPtr(ByVal strOb)
Let strOb = "Alan"
Debug.Print VarPtr(strOb), VarPtr(ByVal strOb)
Let strOb = String(99999999, "x")
Debug.Print VarPtr(strOb), VarPtr(ByVal strOb)
End Sub
The first number in all the output pairs should never change as that is the number held in the COFF symbol table.
In the first 3 character cases the second number will either never change or just change slightly
For the last output line, the second number will very likely change, and possibly a lot. This is because the extremely large string may require VBA / your computer to find some place perhaps less often used for such things: Given that strings can be up to a GByte or two, some flexibility is required in organising their storage. (As ever the actual numbers obtained will vary depending on when / where the coding is done)
_._______________________________________---
A lot was covered in this post, and an important concept, the zero length string was. We are about half way in the total coding and are at a convenient break point as the next thing dealt with will be more detail to the briefly mentioned vbNullString….
Bookmarks